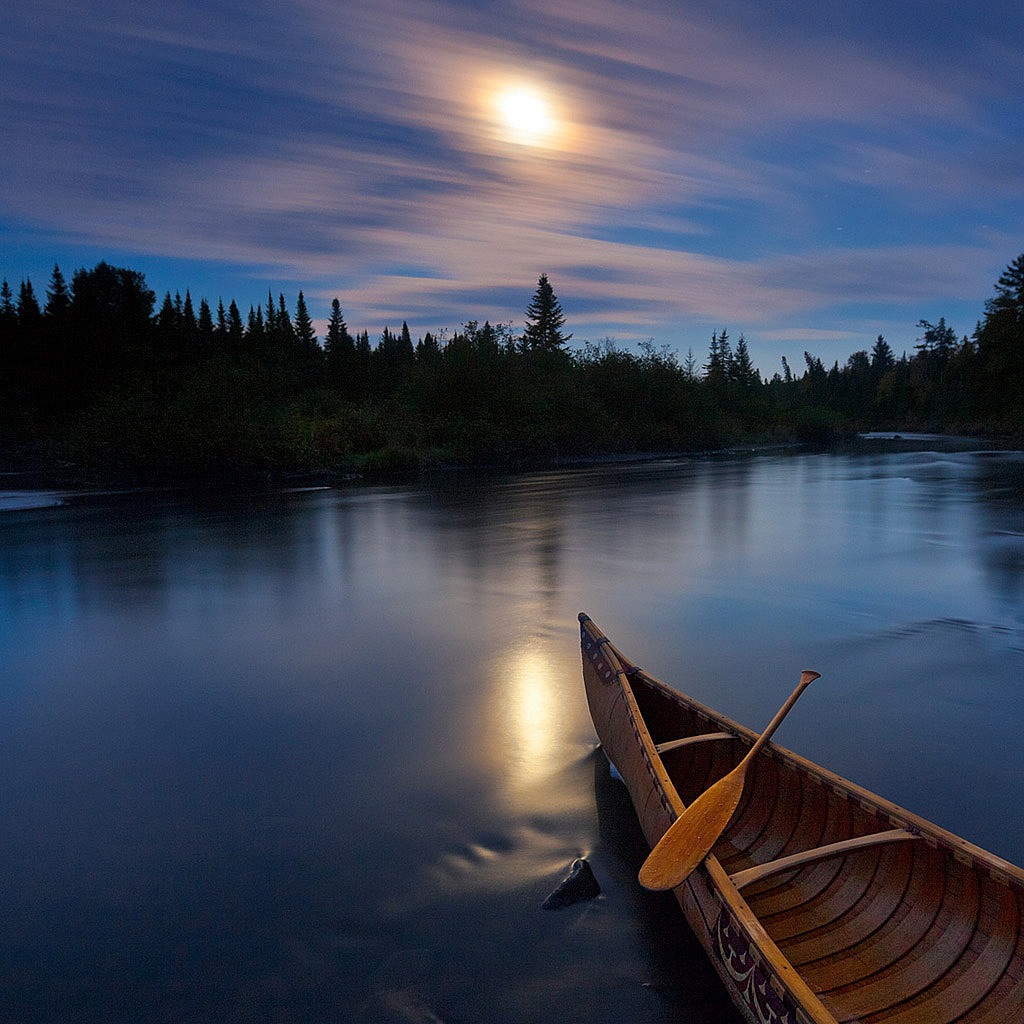
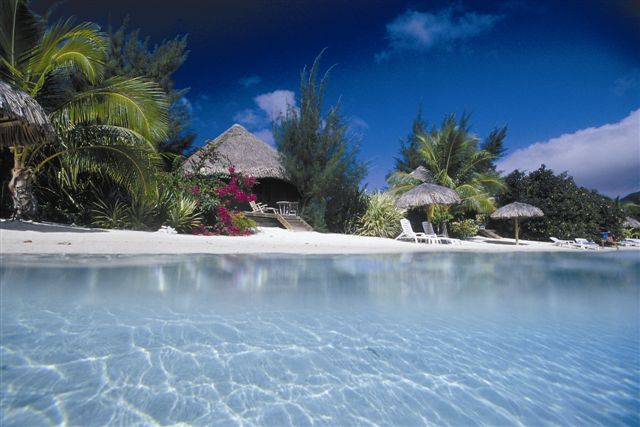
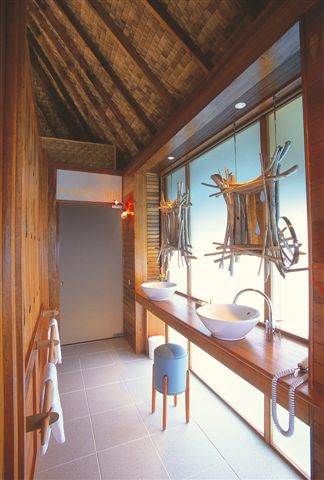
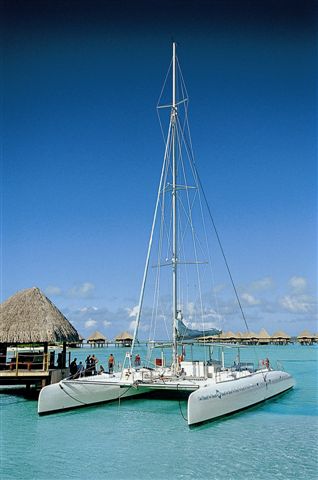
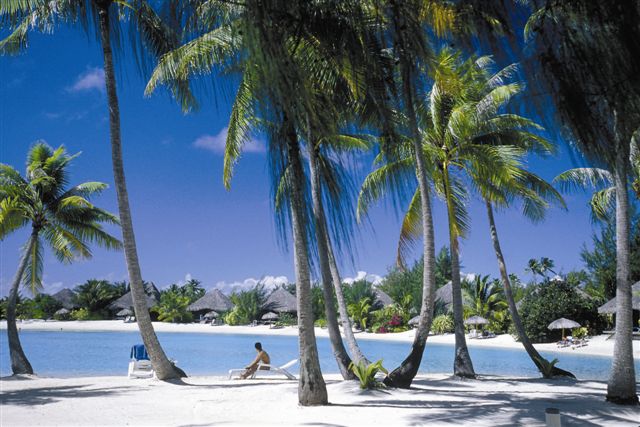
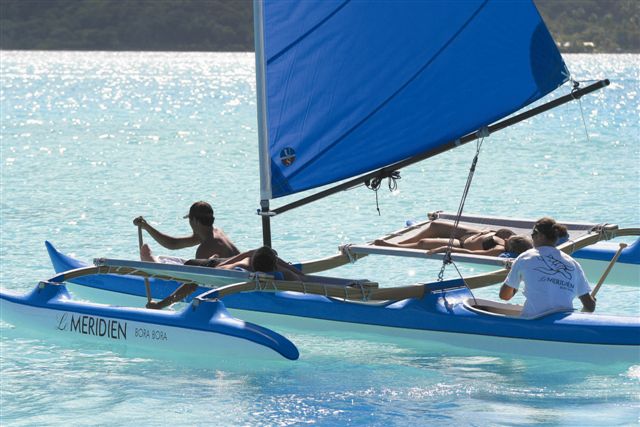
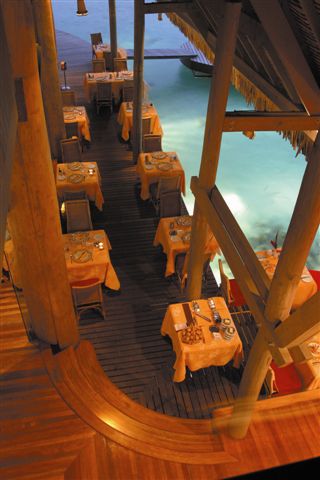
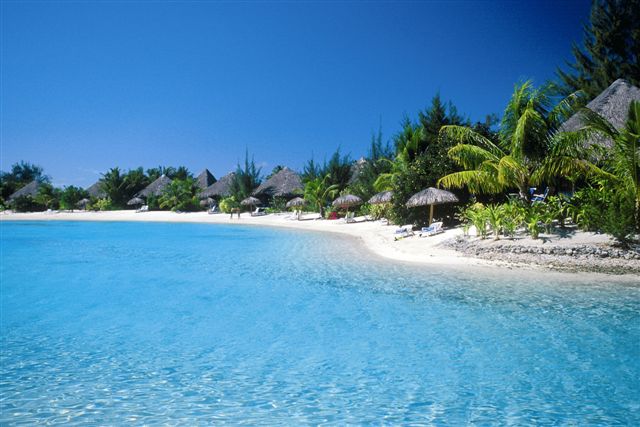
revision: December 15, 2024
enlarge pictures in a gallery
<div> <div class="gallery" onclick="openLightbox(event)"> <img src="../images/1.jpg" alt="Image 1"> <img src="../images/2.jpg" alt="Image 2"> <img src="../images/3.jpg" alt="Image 3"> <img src="../images/4.jpg" alt="Image 4"> </div> <!-- Lightbox container --> <div id="lightbox"> <!-- Close button --> <span id="close-btn" onclick="closeLightbox()">×</span> <!-- Main lightbox image --> <img id="lightbox-img" src="" alt="lightbox image"> <!-- Thumbnails container --> <div id="thumbnail-container"> <!-- Thumbnails will be added dynamically using JavaScript --> </div> <!-- Previous and Next buttons --> <button id="prev-btn" onclick="changeImage(-1)">< Prev</button> <button id="next-btn" onclick="changeImage(1)">Next ></button> </div> </div> <style> .gallery img { margin: 10px; cursor: pointer; max-width: 300px; width: 50%; height: 50%; border-radius: 10px;} /* Lightbox styles */ #lightbox {display: none; position: fixed; top: 0;left: 0; width: 100%; height: 100%; background: rgba(0, 0, 0, 0.8); justify-content: center; align-items: center; overflow: hidden; flex-direction: column; } #lightbox img { max-width: 80%; max-height: 60vh; box-shadow: 0 0 25px rgba(0, 0, 0, 0.8); border-radius: 10px;} #close-btn {position: absolute; top: 10px; right: 10px; font-size: 24px; color: #fff; cursor: pointer; z-index: 2; } /* Style for navigation buttons */ #prev-btn, #next-btn { position: absolute; top: 50%; transform: translateY(-50%); font-size: 20px; color: #fff; background-color: rgba(0, 0, 0, 0.5); border: none; padding: 10px; cursor: pointer; transition: background-color 0.3s;} #prev-btn {left: 10px; } #next-btn { right: 10px;} #prev-btn:hover, #next-btn:hover {background-color: rgba(0, 0, 0, 0.8); } /* Styles for thumbnails */ .thumbnail-container {display: flex; flex-direction: row; flex-wrap: wrap; justify-content: center; } .thumbnail {max-width: 50px; width: 100px; cursor: pointer; margin-top: 40px; margin-left: 5px; margin-right: 5px; border: 2px solid #fff; transition: opacity 0.3s; } .thumbnail:hover, .thumbnail.active-thumbnail {opacity: 0.7;} </style> <script> let currentIndex = 0; const images = document.querySelectorAll('.gallery img'); const totalImages = images.length; // Open the lightbox function openLightbox(event) { if (event.target.tagName === 'IMG') { const clickedIndex = Array.from(images).indexOf(event.target); currentIndex = clickedIndex; updateLightboxImage(); document.getElementById('lightbox').style.display = 'flex'; } } // Close the lightbox function closeLightbox() { document.getElementById('lightbox').style.display = 'none'; } // Change the lightbox image based on direction (1 for next, -1 for prev) function changeImage(direction) { currentIndex += direction; if (currentIndex >= totalImages) { currentIndex = 0; } else if (currentIndex < 0) { currentIndex = totalImages - 1; } updateLightboxImage(); } // Update the lightbox image and thumbnails function updateLightboxImage() { const lightboxImg = document.getElementById('lightbox-img'); const thumbnailContainer = document.getElementById('thumbnail-container'); // Update the main lightbox image lightboxImg.src = images[currentIndex].src; // Clear existing thumbnails thumbnailContainer.innerHTML = ''; // Add new thumbnails images.forEach((image, index) => { const thumbnail = document.createElement('img'); thumbnail.src = image.src; thumbnail.alt = `Thumbnail ${index + 1}`; thumbnail.classList.add('thumbnail'); thumbnail.addEventListener('click', () => updateMainImage(index)); thumbnailContainer.appendChild(thumbnail); }); // Highlight the current thumbnail const thumbnails = document.querySelectorAll('.thumbnail'); thumbnails[currentIndex].classList.add('active-thumbnail'); } // Update the main lightbox image when a thumbnail is clicked function updateMainImage(index) { currentIndex = index; updateLightboxImage(); } // Add initial thumbnails updateLightboxImage(); // To add keyboard navigation (left/right arrow keys) document.addEventListener('keydown', function (e) { if (document.getElementById('lightbox').style.display === 'flex') { if (e.key === 'ArrowLeft') { changeImage(-1); } else if (e.key === 'ArrowRight') { changeImage(1); } } }); </script>
artistic picture gallery (without JavaScript)
<article class="grid-gallery"> <img src="../images/1.jpg" alt="description of picture 1" /> <img src="../images/2.jpg" alt="description of picture 2" /> <img src="../images/3.jpg" alt="description of picture 3" /> <img src="../images/4.jpg" alt="description of picture 4" /> <img src="../images/5.jpg" alt="description of picture 5" /> <img src="../images/6.jpg" alt="description of picture 6" /> <img src="../images/7.jpg" alt="description of picture 7" /> <img src="../images/8.jpg" alt="description of picture 8" /> </article> <style> .grid-gallery { --size: 100px; display: grid; grid-template-columns: repeat(6, var(--size)); grid-auto-rows: var(--size); gap: 5px; place-items: start center; margin-bottom: var(--size);} .grid-gallery img { width: calc(var(--size) * 2); height: calc(var(--size) * 2); object-fit: cover; grid-column: auto / span 2; border-radius: 5px; clip-path: path("M90,10 C100,0 100,0 110,10 190,90 190,90 190,90 200,100 200,100 190,110 190,110 110,190 110,190 100,200 100,200 90,190 90,190 10,110 10,110 0,100 0,100 10,90Z"); } .grid-gallery img:nth-child(5n - 1) { grid-column: 2 / span 2;} .grid-gallery:has(img:hover) img:not(:hover) {filter: brightness(0.5) contrast(0.5);} .grid-gallery img { /* ... */ transition: clip-path 0.25s, filter 0.75s;} .grid-gallery img:hover { clip-path: path("M0,0 C0,0 200,0 200,0 200,0 200,100 200,100 200,100 200,200 200,200 200,200 100,200 100,200 100,200 100,200 0,200 0, 200 0,100 0,100 0,100 0,100 0,100Z"); transition: clip-path 0.25s, filter 0.25s; z-index: 1;} grid-gallery a:focus {outline: 1px dashed black; outline-offset: -5px;} </style>
click to open enarge picture below
<div> <!-- The grid: four columns --> <div class="row"> <div class="column"><img src="../images/6.jpg" alt="Shanghai" width="50%" onclick="myFunction(this);"></div> <div class="column"><img src="../images/7.jpg" alt="Shanghai" width="50%" onclick="myFunction(this);"></div> <div class="column"><img src="../images/8.jpg" alt="Shanghai" width="50%" onclick="myFunction(this);"></div> <div class="column"><img src="../images/9.jpg" alt="Shanghai" width="50%" onclick="myFunction(this);"></div> </div> <!-- The expanding image container --> <div class="container"> <!-- Close the image --> <span onclick="this.parentElement.style.display='none'" class="closebtn">×</span> <!-- Expanded image --> <img id="expandedImg" style="width:80%"> <!-- Image text --> <div id="imgtext"></div> </div> </div> <style> /* The grid: Four equal columns that floats next to each other */ .column {float: left; width: 25%; padding: 10px; } /* Style the images inside the grid */ .column img {opacity: 0.8; cursor: pointer;} .column img:hover { opacity: 1;} /* Clear floats after the columns */ .row:after {content: ""; display: table; clear: both;} /* The expanding image container (positioning is needed to position the close button and the text) */ .container {position: relative; display: none;} /* Expanding image text */ #imgtext {position: absolute; bottom: 15px; left: 15px; color: white; font-size: 20px;} /* Closable button inside the image */ .closebtn {position: absolute; top: 10px; right: 25px; color: blue; font-size: 35px; cursor: pointer; } </style> <script> function myFunction(imgs) { // Get the expanded image var expandImg = document.getElementById("expandedImg"); // Get the image text var imgText = document.getElementById("imgtext"); // Use the same src in the expanded image as the image being clicked on from the grid expandImg.src = imgs.src; // Use the value of the alt attribute of the clickable image as text inside the expanded image imgText.innerHTML = imgs.alt; // Show the container element (hidden with CSS) expandImg.parentElement.style.display = "block"; } </script>
enlarge picture by clicking it
<div> <!-- Trigger the Modal --> <img id="myImg" src="../images/12.jpg" alt="Shanghai" style="width:100%;max-width:300px"> <!-- The Modal --> <div id="myModal" class="modal"> <!-- The Close Button --> <span class="close">×</span> <!-- Modal Content (The Image) --> <img class="modal-content" id="img01"> <!-- Modal Caption (Image Text) --> <div id="caption"></div> </div> </div> <style> /* Style the Image Used to Trigger the Modal */ #myImg {border-radius: 5px; cursor: pointer; transition: 0.3s; } #myImg:hover {opacity: 0.7;} /* The Modal (background) */ .modal {display: none; position: fixed; z-index: 1; padding-top: 100px; left: 0; top: 0; width: 100%; height: 100%; overflow: auto; /* Enable scroll if needed */ background-color: rgb(0,0,0); background-color: rgba(0,0,0,0.9); } /* Modal Content (Image) */ .modal-content {margin: auto; display: block; width: 80%; max-width: 700px;} /* Caption of Modal Image (Image Text) - Same Width as the Image */ #caption { margin: auto; display: block; width: 80%; max-width: 700px; text-align: center; padding: 10px 0; height: 150px;} /* Add Animation - Zoom in the Modal */ .modal-content, #caption { animation-name: zoom; animation-duration: 0.6s; } @keyframes zoom { from {transform:scale(0)} to {transform:scale(1)} } /* The Close Button */ .close {position: absolute; top: 15px; right: 35px; color: #f1f1f1; font-size: 40px; font-weight: bold; transition: 0.3s;} .close:hover, .close:focus {color: #bbb; text-decoration: none; cursor: pointer; } /* 100% Image Width on Smaller Screens */ @media only screen and (max-width: 700px){ .modal-content { width: 100%; } } </style> <script> // Get the modal var modal = document.getElementById("myModal"); // Get the image and insert it inside the modal - use its "alt" text as a caption var img = document.getElementById("myImg"); var modalImg = document.getElementById("img01"); var captionText = document.getElementById("caption"); img.onclick = function(){ modal.style.display = "block"; modalImg.src = this.src; captionText.innerHTML = this.alt; } // Get the <span> element that closes the modal var span = document.getElementsByClassName("close")[0]; // When the user clicks on <span> (x), close the modal span.onclick = function() { modal.style.display = "none"; } </script>